前面采用docker方式搭建了一个mongodb的集群, 今天采用spring boot方式访问这个集群
本文 参考 Spring Boot中使用MongoDB数据库 内容, 一步一步进行实现,
另外, 本文需要的 mongodb集群是前文搭建的 一个集群, 没有准备好的 可以参考: Docker部署一个MongoDB集群
一。 配置java访问mongodb程序的主机的hosts文件
在前面集群安装中,采用了域名配置3个节点,这样在java的程序的主机上要进行配置
或者这些域名是dns能够正确解析的, 否则就出现如下图的socket连接超时的情况
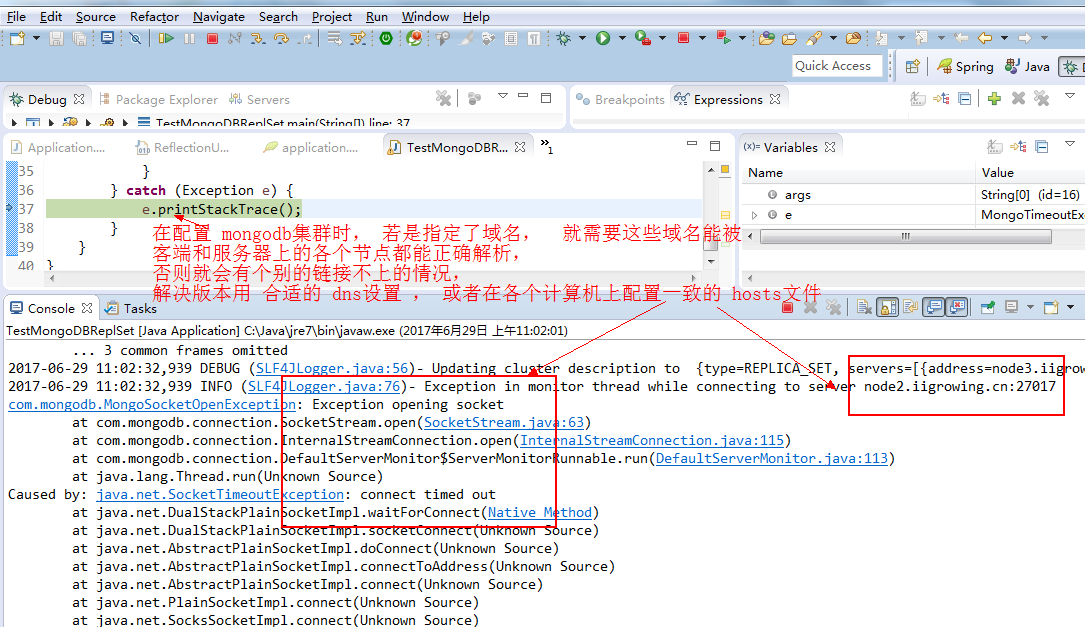
为了解决问题,我们配置了我们的hosts文件图
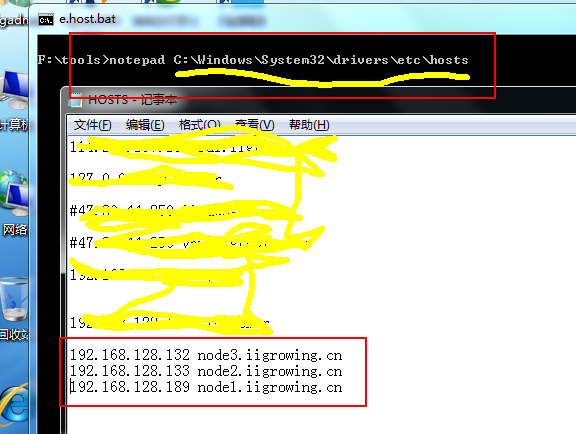
在windows下要用管理员身份进行操作, 否则无法保存
二。 编写java代码访问集群
package cn.iigrowing.study.mongodb; import java.util.ArrayList; import java.util.List; import com.mongodb.BasicDBObject; import com.mongodb.DB; import com.mongodb.DBCollection; import com.mongodb.DBCursor; import com.mongodb.DBObject; import com.mongodb.MongoClient; import com.mongodb.ServerAddress; public class TestMongoDBReplSet { public static void main(String[] args) { try { List<ServerAddress> addresses = new ArrayList<ServerAddress>(); ServerAddress address1 = new ServerAddress("192.168.128.189", 27017); ServerAddress address2 = new ServerAddress("192.168.128.132", 27017); ServerAddress address3 = new ServerAddress("192.168.128.133", 27017); addresses.add(address1); addresses.add(address2); addresses.add(address3); MongoClient client = new MongoClient(addresses); DB db = client.getDB("test"); DBCollection coll = db.getCollection("testdb"); // 插入 BasicDBObject object = new BasicDBObject(); object.append("test2", "testval2"); coll.insert(object); DBCursor dbCursor = coll.find(); while (dbCursor.hasNext()) { DBObject dbObject = dbCursor.next(); System.out.println(dbObject.toString()); } } catch (Exception e) { e.printStackTrace(); } } }
拷贝上面程序到sts中, 运行后会报如下异常
"errmsg" : "not authorized on test to execute command { insert: \"testdb\"
原因是程序里面没有正确配置用户名和密码造成,修改程序里面的用户名和密码
注意:
为了访问mongodb的数据库系统,需要首先在mongo中添加相关的用户和数据库
然后用rockmongo创建数据库, 最后失败, 原因不详, 后来查询获取到在mongodb用 认证模式启动后, 必须超级管理员才能创建数据库,普通用户不能
关于权限信息参考: MongoDB 用户和身份验证
另外, 在副本集这个集群模式中, 也必须在PRIMARY这个集群上才能创建。
由于对mongo了解的不多, 因此只好登录mongo的主节点进行操作
在mongodb的主的 机器中 运行 docker exec -t -i mongo bash
进入了容器里面, 然后运行mongo命令进入控制台, 如下:
[root@my03 rockmongo]# docker exec -t -i mongo bash
root@node3:/# mongo
MongoDB shell version: 2.6.5
connecting to: test
rs0:PRIMARY>
rs0:PRIMARY>
然后用下面命令登录 rs0:PRIMARY> db.auth("siteRootAdmin","password");
1
用下面命令创建数据库
rs0:PRIMARY> use mytest03;
switched to db mytest03
用下面命令 创建一个用户
rs0:PRIMARY> db.createUser( {
... user: "mytest03",
... pwd: "password",
... roles: [ { role: "dbOwner", db: "mytest03" } ]
... });
Successfully added user: {
"user" : "mytest03",
"roles" : [
{
"role" : "dbOwner",
"db" : "mytest03"
}
]
}
用下面命令登录
rs0:PRIMARY> db.auth("mytest03","password");
1
最后在修改java代码添加数据库的用户名和密码
下面是从网络上搜索到的 访问mongodb时的java例子
Java Code Examples for com.mongodb.MongoCredential The following are top voted examples for showing how to use com.mongodb.MongoCredential. These examples are extracted from open source projects. You can vote up the examples you like and your votes will be used in our system to product more good examples. + Save this class to your library Example 1 Project: Trivial4b File: TrivialAPI.java View source code 9 votes vote down vote up private static DB conectar() { MongoClient mongoClient = null; MongoCredential mongoCredential = MongoCredential .createMongoCRCredential("trivialuser", "trivial", "4btrivialmongouser".toCharArray()); try { mongoClient = new MongoClient(new ServerAddress( "ds062797.mongolab.com", 62797), Arrays.asList(mongoCredential)); } catch (UnknownHostException e) { e.printStackTrace(); } DB db = mongoClient.getDB("trivial"); System.out.println("Conexion creada con la base de datos"); return db; } Example 2 Project: ff4j File: FeatureStoreMongoCollectionCore1Test.java View source code 7 votes vote down vote up /** * Open real connection to MongoDB. * * @return * target mongo client * @throws UnknownHostException * exeption when creating server address */ private MongoClient getMongoClient() throws UnknownHostException { // Given (using real connection) MongoCredential credential = MongoCredential.createMongoCRCredential("username", "FF4J", "password".toCharArray()); ServerAddress adr = new ServerAddress("localhost", 22012); return new MongoClient(adr, Arrays.asList(credential)); } Example 3 Project: deep-spark File: MongoReader.java View source code 6 votes vote down vote up /** * Init void. * * @param partition the partition */ public void init(Partition partition) { try { List<ServerAddress> addressList = new ArrayList<>(); for (String s : (List<String>) ((DeepPartition) partition).splitWrapper().getReplicas()) { addressList.add(new ServerAddress(s)); } //Credentials List<MongoCredential> mongoCredentials = new ArrayList<>(); if (mongoDeepJobConfig.getUsername() != null && mongoDeepJobConfig.getPassword() != null) { MongoCredential credential = MongoCredential.createMongoCRCredential(mongoDeepJobConfig.getUsername(), mongoDeepJobConfig.getDatabase(), mongoDeepJobConfig.getPassword().toCharArray()); mongoCredentials.add(credential); } mongoClient = new MongoClient(addressList, mongoCredentials); mongoClient.setReadPreference(ReadPreference.valueOf(mongoDeepJobConfig.getReadPreference())); db = mongoClient.getDB(mongoDeepJobConfig.getDatabase()); collection = db.getCollection(mongoDeepJobConfig.getCollection()); dbCursor = collection.find(generateFilterQuery((MongoPartition) partition), mongoDeepJobConfig.getDBFields()); } catch (UnknownHostException e) { throw new DeepExtractorInitializationException(e); } } Example 4 Project: opensearchserver File: DatabaseCrawlMongoDb.java View source code 6 votes vote down vote up MongoClient getMongoClient() throws URISyntaxException, UnknownHostException { String user = getUser(); String password = getPassword(); URI uri = new URI(getUrl()); MongoCredential credential = null; if (!StringUtils.isEmpty(user) && !StringUtils.isEmpty(password)) { credential = MongoCredential.createMongoCRCredential(user, databaseName, password.toCharArray()); return new MongoClient(new ServerAddress(uri.getHost(), uri.getPort()), Arrays.asList(credential)); } return new MongoClient(new ServerAddress(uri.getHost(), uri.getPort())); } Example 5 Project: zest-java File: MongoMapEntityStoreMixin.java View source code 6 votes vote down vote up @Override public void activateService() throws Exception { loadConfiguration(); // Create Mongo driver and open the database if( username.isEmpty() ) { mongo = new MongoClient( serverAddresses ); } else { MongoCredential credential = MongoCredential.createMongoCRCredential( username, databaseName, password ); mongo = new MongoClient( serverAddresses, Arrays.asList( credential ) ); } db = mongo.getDB( databaseName ); // Create index if needed db.requestStart(); DBCollection entities = db.getCollection( collectionName ); if( entities.getIndexInfo().isEmpty() ) { entities.createIndex( new BasicDBObject( IDENTITY_COLUMN, 1 ) ); } db.requestDone(); } Example 6 Project: XBDD File: ServletContextMongoClientFactory.java View source code 6 votes vote down vote up private MongoDBAccessor getMongoDBAccessor() throws UnknownHostException { final MongoClient mc; if (this.username != null) { MongoCredential credentials = MongoCredential.createMongoCRCredential(this.username, "admin", this.password); mc = new MongoClient(new ServerAddress(this.host, this.port), Arrays.asList(credentials)); } else { mc = new MongoClient(this.host, this.port); } return new MongoDBAccessor(mc); } Example 7 Project: spacesimulator File: MongoClientTest.java View source code 6 votes vote down vote up @Test public void herokuTest() throws UnknownHostException { MongoCredential credential = MongoCredential.createMongoCRCredential("heroku_app25459577", "heroku_app25459577", "gsps2dbe8l7ovbot1jkto5lnid".toCharArray()); MongoClient mongoClient = new MongoClient(new ServerAddress("ds041841.mongolab.com" , 41841), Arrays.asList(credential)); MongoDatabase db = mongoClient.getDatabase("heroku_app25459577"); ListCollectionsIterable<Document> list = db.listCollections(); System.out.println(list.toString()); mongoClient.close(); } Example 8 Project: metric File: DashboardApi.java View source code 6 votes vote down vote up @Override public void init() throws ServletException { try { Properties p = Conf.load("Mongo"); ServerAddress addr = new ServerAddress(p.getProperty("host"), Numbers.parseInt(p.getProperty("port"), 27017)); String database = p.getProperty("db"); String username = p.getProperty("username"); String password = p.getProperty("password"); if (username == null || password == null) { mongo = new MongoClient(addr); } else { mongo = new MongoClient(addr, Collections.singletonList(MongoCredential. createMongoCRCredential(username, database, password.toCharArray()))); } db = mongo.getDB(database); maxTagValues = Numbers.parseInt(Conf. load("Dashboard").getProperty("max_tag_values")); } catch (IOException e) { throw new ServletException(e); } } Example 9 Project: incubator-brooklyn File: MongoDBClientSupport.java View source code 6 votes vote down vote up private MongoClient baseClient(MongoClientOptions connectionOptions) { if (usesAuthentication) { MongoCredential credential = MongoCredential.createMongoCRCredential(username, authenticationDatabase, password.toCharArray()); return new MongoClient(address, ImmutableList.of(credential), connectionOptions); } else { return new MongoClient(address, connectionOptions); } } Example 10 Project: muikku File: MongoLogProvider.java View source code 6 votes vote down vote up @PostConstruct public void init() { String user = pluginSettingsController.getPluginSetting("mongo-log", "database.user"); String password = pluginSettingsController.getPluginSetting("mongo-log", "database.password"); String host = pluginSettingsController.getPluginSetting("mongo-log", "database.host"); String port = pluginSettingsController.getPluginSetting("mongo-log", "database.port"); String database = pluginSettingsController.getPluginSetting("mongo-log", "database.name"); try { if (StringUtils.isNotBlank(user) && StringUtils.isNotBlank(password) && StringUtils.isNumeric(port) && StringUtils.isNotBlank(host) && StringUtils.isNotBlank(database)) { MongoCredential credential = MongoCredential.createMongoCRCredential(user, database, password.toCharArray()); ServerAddress addr = new ServerAddress(host, NumberUtils.createInteger(port)); mongo = new MongoClient(addr, Arrays.asList(credential)); db = mongo.getDB(database); } else { logger.warning("Could not initialize mongo log because some of the settings were missing"); } } catch (Exception e) { logger.warning("Cannot initialize connection to mongoDB"); enabled = false; } } Example 11 Project: opencga File: MongoCredentials.java View source code 6 votes vote down vote up public MongoCredentials(String mongoHost, int mongoPort, String mongoDbName, String mongoUser, String mongoPassword) throws IllegalOpenCGACredentialsException { dataStoreServerAddresses = new LinkedList<>(); dataStoreServerAddresses.add(new DataStoreServerAddress(mongoHost, mongoPort)); this.mongoDbName = mongoDbName; if (mongoUser != null && mongoPassword != null) { mongoCredentials = MongoCredential.createMongoCRCredential(mongoUser, mongoDbName, mongoPassword.toCharArray()); } check(); } Example 12 Project: IPM_MediaCenter File: Database.java View source code 6 votes vote down vote up private Database() { MongoCredential credential = MongoCredential.createMongoCRCredential(SettingsManager.getSetting("mongo", "user"), SettingsManager.getSetting("mongo", "authentication_db"), SettingsManager.getSetting("mongo", "password").toCharArray()); try { mongo = new MongoClient(new ServerAddress(SettingsManager.getSetting("mongo", "server"), SettingsManager.getIntegerSetting("mongo", "port")), Arrays.asList(credential)); morphia = new Morphia(); ds = morphia.createDatastore(mongo, SettingsManager.getSetting("mongo", "database")); ds.ensureIndexes(); } catch (UnknownHostException e) { e.printStackTrace(); } } Example 13 Project: SEAS File: RestrictedService.java View source code 6 votes vote down vote up public void init() throws ServletException { if(!gateInited) { try { // GATE home is setted, so it can be used by SAGA. ServletContext ctx = getServletContext(); File gateHome = new File(ctx.getRealPath("/WEB-INF")); Gate.setGateHome(gateHome); // GATE user configuration file is setted. Gate.setUserConfigFile(new File(gateHome, "user-gate.xml")); //GATE is initialized as non visible. Gate.init(); // We load the plugins that are going to be used by the SAGA modules. // Load ANNIE. Gate.getCreoleRegister().registerDirectories( ctx.getResource("/WEB-INF/plugins/ANNIE")); // Load processingResources (from SAGA) Gate.getCreoleRegister().registerDirectories( ctx.getResource("/WEB-INF/plugins/processingResources")); // Load webProcessingResources (from SAGA) Gate.getCreoleRegister().registerDirectories( ctx.getResource("/WEB-INF/plugins/webProcessingResources")); // Now we create the sentiment analysis modules that are going to be provided by the service. // English financial module. ArrayList<URL> dictionaries = new ArrayList<URL>(); dictionaries.add((new RestrictedService()).getClass().getResource("/LoughranMcDonald/lists.def")); modules.put("enFinancial", new DictionaryBasedSentimentAnalyzer("SAGA - Sentiment Analyzer", dictionaries)); // English financial + emoticon module. ArrayList<URL> dictionaries2 = new ArrayList<URL>(); dictionaries2.add((new RestrictedService()).getClass().getResource("/LoughranMcDonald/lists.def")); dictionaries2.add((new RestrictedService()).getClass().getResource("/resources/gazetteer/emoticon/lists.def")); modules.put("enFinancialEmoticon", new DictionaryBasedSentimentAnalyzer("SAGA - Sentiment Analyzer", dictionaries2)); // Mongo login String user = ""; String password = ""; MongoCredential credential = MongoCredential.createMongoCRCredential(user,"RestrictedDictionaries",password.toCharArray()); MongoClient mongoClient = new MongoClient(new ServerAddress("localhost"), Arrays.asList(credential)); db = mongoClient.getDB("RestrictedDictionaries"); gateInited = true; } catch(Exception ex) { throw new ServletException("Exception initialising GATE", ex); } } } Example 14 Project: Duke File: MongoDBDataSource.java View source code 5 votes vote down vote up public RecordIterator getRecords() { verifyProperty(dbname, "database"); verifyProperty(collectionName, "collection"); try { final MongoClient mongo; final DB database; final DBCollection collection; final DBCursor result; final DBObject queryDocument; final DBObject projectionDocument; // authentication mecanism via MONGODB-CR authentication http://docs.mongodb.org/manual/core/authentication/#authentication-mongodb-cr if(auth.equals(AUTH_ON_DB)){ verifyProperty(username, "user-name"); verifyProperty(password, "password"); mongo = new MongoClient( new ServerAddress(mongouri, port), Arrays.asList(MongoCredential.createMongoCRCredential(username, dbname, password.toCharArray())) ); } else if(auth.equals(AUTH_ON_ADMIN)){ verifyProperty(username, "user-name"); verifyProperty(password, "password"); mongo = new MongoClient( new ServerAddress(mongouri, port), Arrays.asList(MongoCredential.createMongoCRCredential(username, AUTH_ON_ADMIN, password.toCharArray())) ); } else{ mongo = new MongoClient(new ServerAddress(mongouri, port)); } // get db, collection database = mongo.getDB(dbname); collection = database.getCollection(collectionName); // execute query queryDocument = (DBObject)JSON.parse(query); if(projection==null){ result = collection.find(queryDocument); } else{ projectionDocument = (DBObject)JSON.parse(projection); result = collection.find(queryDocument, projectionDocument); } // See: http://api.mongodb.org/java/current/com/mongodb/DBCursor.html#addOption(int) // and http://api.mongodb.org/java/current/com/mongodb/Bytes.html#QUERYOPTION_NOTIMEOUT if(noTimeOut){ result.addOption(Bytes.QUERYOPTION_NOTIMEOUT); } return new MongoDBIterator(result, mongo); } catch (UnknownHostException e) { throw new RuntimeException(e); } catch (Exception ex){ throw new DukeException(ex); } } Example 15 Project: MedtronicUploader File: MedtronicCGMService.java View source code 5 votes vote down vote up /** * This method initializes only one instance of mongo db (It would be better use something like Google guice but ...) * @return DB initialized successfully */ private boolean initializeDB(){ dbURI = prefs.getString("MongoDB URI", null); collectionName = prefs.getString("Collection Name", "entries"); dsCollectionName = prefs.getString("DeviceStatus Collection Name", "devicestatus"); gdCollectionName = prefs.getString("gcdCollectionName", null); devicesCollectionName = "devices"; db = null; if (dbURI != null) { log.debug("URI != null"); try { if (!prefs.getBoolean("isMongoRest", false)){ // connect to db gYumpKyCgbOhcAGOTXvkCcq4V04W6K1Z MongoClientURI uri = new MongoClientURI(dbURI.trim()); Builder b = MongoClientOptions.builder(); b.heartbeatConnectTimeout(150000); b.heartbeatFrequency(120000); b.heartbeatSocketTimeout(150000); b.maxWaitTime(150000); b.connectTimeout(150000); boolean bAchieved = false; String user = ""; String password = ""; String source = ""; String host = ""; String port = ""; int iPort = -1; if (dbURI.length() > 0){ String[] splitted = dbURI.split(":"); if (splitted.length >= 4 ){ user = splitted[1].substring(2); if (splitted[2].indexOf("@") < 0) bAchieved = false; else{ password = splitted[2].substring(0,splitted[2].indexOf("@")); host = splitted[2].substring(splitted[2].indexOf("@")+1, splitted[2].length()); if (splitted[3].indexOf("/") < 0) bAchieved = false; else{ port = splitted[3].substring(0, splitted[3].indexOf("/")); source = splitted[3].substring(splitted[3].indexOf("/")+1, splitted[3].length()); try{ iPort = Integer.parseInt(port); }catch(Exception ne){ iPort = -1; } if (iPort > -1) bAchieved = true; } } } } log.debug("Uri TO CHANGE user "+user+" host "+source+" password "+password); if (bAchieved){ MongoCredential mc = MongoCredential.createMongoCRCredential(user, source , password.toCharArray()); ServerAddress sa = new ServerAddress(host, iPort); List<MongoCredential> lcredential = new ArrayList<MongoCredential>(); lcredential.add(mc); if (sa != null && sa.getHost() != null && sa.getHost().indexOf("localhost") < 0){ client = new MongoClient(sa, lcredential, b.build()); } } // get db db = client.getDatabase(uri.getDatabase()); // get collection dexcomData = null; glucomData = null; deviceData = db.getCollection(devicesCollectionName); if (deviceData == null){ db.createCollection("device", null); deviceData = db.getCollection("device"); } if (collectionName != null) dexcomData = db.getCollection(collectionName.trim()); if (gdCollectionName != null) glucomData = db.getCollection(gdCollectionName.trim()); dsCollection = db.getCollection(dsCollectionName); if (dsCollection == null){ db.createCollection("devicestatus", null); dsCollection = db.getCollection("devicestatus"); } } }catch (Exception e){ log.error("EXCEPTION INIT",e); return false; } return true; } return false; }
选取合适的代码放置到我们的代码中, 代码如下:
MongoCredential credential = MongoCredential.createMongoCRCredential("mytest03", "mytest03", "password".toCharArray());
MongoClient client = new MongoClient(addresses, Arrays.asList(credential));
然后运行程序, 成功访问mongodb
最后在 rockmongo中查看数据添加成功